Chat UI Components
Before you can use these UI Components, please do the basic setup.
<ChatMessageBell/>
Description
The <ChatMessageBell/>
component is typically used in the header off your application. It informs the user about
unread messages in the chat. Please refer to the chat feature guide for more information and
instructions.
All the Chat related UI widgets need to be used within a <ChatProvider>
component.
The <ChatProvider>
needs to be inserted only once, at a higher level component, typically in the App.tsx or a layout component.
The <ChatMessageBell />
requires the <Chat/>
component to be used as well.
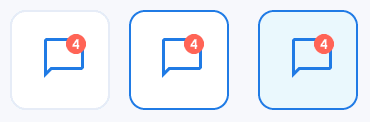
Integration
To show the bell component, you can copy this snippet in your code:
import { ChatProvider, ChatMessageBell } from '@roq/ui-react';
function App() {
return (
<ChatProvider>
<TopBar>
<ChatMessageBell />
</TopBar>
</ChatProvider>
);
}
<Chat/>
Description
The <Chat/>
UI component represents the chat message center with sidebar and the chat window. Please refer to
the chat feature guide for more information and instructions.
Alternatively to the <Chat/>
component, you can also use the individuals parts: <ChatWindow/>
and
<ChatSidebar/>
, see integration guidelines below.
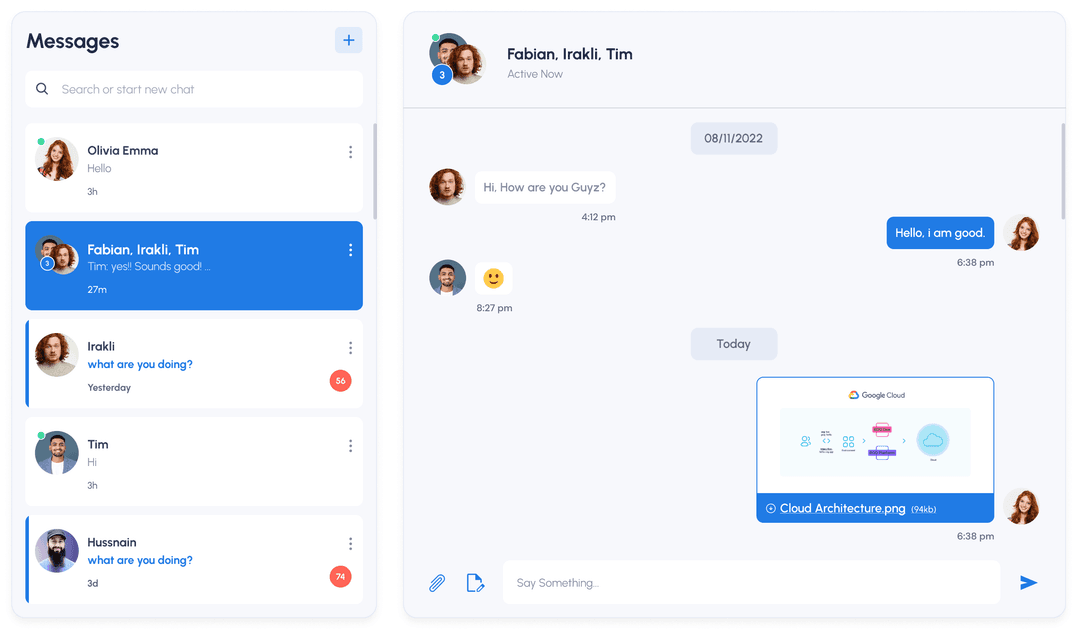
Integration
All the Chat related UI widgets need to be used within a <ChatProvider>
component.
The <ChatProvider>
needs to be inserted only once, at a higher level component, typically in the App.tsx or a layout component.
<ChatProvider/>
The ChatProvider component is a wrapper that provides a context to it's child components. Typically it is present on a root or layout component.
It accepts several configuration options:
import { ChatProvider, Chat } from '@roq/ui-react';
function App() {
return (
<ChatProvider
enableGroups={false}
enableFiles={false}
enableFormatting={false}
enableForwarding={false}
>
<Component />
</ChatProvider>
) }
<Chat/>
You can place the entire chat on your page using this snippet:
import { ChatProvider, Chat } from '@roq/ui-react';
function ChatPage() {
return (
<ChatProvider>
<Chat />
</ChatProvider>
)
}
//You can optionally filter chat conversations by supplying the "tags" prop
function ChatPage() {
return (
<ChatProvider>
<Chat tags={["hiring", "hackathons"]} />
</ChatProvider>
)
}
<ChatWindow/>
In case you only want to show the chat conversation window, then use this component.
import { ChatProvider, ChatWindow } from "@roq/ui-react";
function ChatPage({ conversationId }) {
return (
<ChatProvider>
<ChatWindow conversationId={conversationId} />
</ChatProvider>
);
}
<ChatSidebar/>
You can show just the conversation list by using this component.
import { ChatProvider, ChatSidebar } from "@roq/ui-react";
function ChatPage() {
return (
<ChatProvider>
<ChatSidebar />
</ChatProvider>
);
}
<ChatSidebar/>
with controlled state: Custom routing example
You can use the <ChatSidebar />
in a controlled state, for example if you would like to implement custom routing when a conversation is selected from it.
In the example below, you
import { useState } from "react";
import { ChatProvider, ChatSidebar } from "@roq/ui-react";
function ChatPage() {
const handleActiveConversationChange = (conversationId: string) => {
// Implement routing based on the react router that you use
window.location.href = `/conversation/${conversationId}`;
};
return (
<ChatProvider>
<ChatSidebar
onCurrentConversationIdChanged={handleActiveConversationChange}
generateConversationLink={(conversation) =>
`/chat?conversation={conversation.id}`
}
/>
</ChatProvider>
);
}
<ChatSidebar/>
and <Chat/>
with conversations filtered by tags
You can supply the "tags" prop to the <ChatSidebar />
or <Chat/>
components to filter conversations that have those specific tags.
The tags can be assigned using a server-side request using GraphQL or our backend SDKs
import { useState } from "react";
import { ChatProvider, ChatSidebar, Chat } from "@roq/ui-react";
function ChatPage() {
const handleActiveConversationChange = (conversationId: string) => {
// Implement routing based on the react router that you use
window.location.href = `/conversation/${conversationId}`;
};
return (
<ChatProvider>
<Chat tags={["hackathons", "hiring"]} />
{/* OR */}
<ChatSidebar tags={["hackathons", "hiring"]} />
</ChatProvider>
);
}
For the usage of <Chat/>
component in Next.js you can see this tutorial page.